15 New project, GitHub first
We create a new Project, with the preferred “GitHub first, then RStudio” sequence.
Why do we prefer this?
Because this method of copying the Project from GitHub to your computer also sets up the local Git repository for immediate pulling and pushing.
Under the hood, we are doing git clone
.
You’ve actually done this before during set up (chapter 12). We’re doing it again, with feeling.
The workflow is pretty similar for other repository managers like GitLab or Bitbucket. We will specify below when you may need to do something differently.
15.1 Make a repo on GitHub
Go to https://github.com and make sure you are logged in.
Near “Repositories”, click the big green “New” button. Or, if you are on your own profile page, click on “Repositories”, then click the big green “New” button.
How to fill this in:
- Repository template: No template.
- Repository name:
myrepo
or whatever you wish to name your new project. Approach this similar to a variable name, in code: descriptive but brief, no whitespace. Letters, digits,-
,.
, or_
are allowed. - Description: “Analysis of the stuff” or any short description of the project. Write this for humans.
- Public.
- Initialize this repository with: Add a README file.
Click the big green button that says “Create repository”.
Now click the big green button that says “<> Code”.
Copy a clone URL to your clipboard. If you’re taking our default advice, copy the HTTPS URL. But if you’re opting for SSH, then make sure to copy the SSH URL.
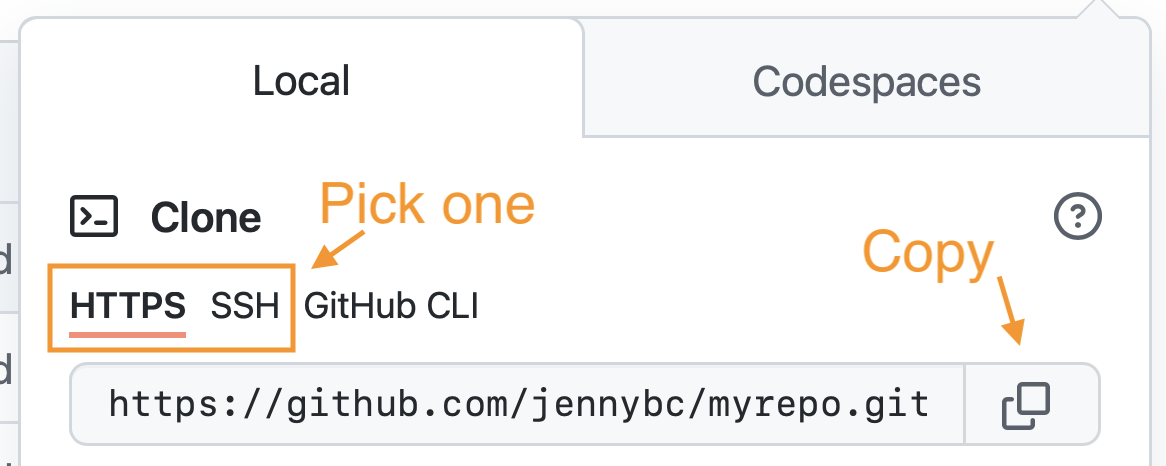
15.1.1 GitLab
Log in at https://gitlab.com. Click on the “+” button in the top-right corner, and then on “New project”.
- Project name:
myrepo
(or whatever you wish)
- Public
- YES Initialize repository with a README
Click the big green button “Create project.”
Copy the HTTPS or SSH clone URL to your clipboard via the blue “Clone” button.
15.1.2 Bitbucket
Log in at https://bitbucket.org. On the left-side pane, click on the “+” button, and then on “Repository” under “Create”.
- Repository name:
myrepo
(or whatever you wish) - Access level: Uncheck to make the repository public.
- Include a README?: Select either “Yes, with a tutorial (for beginners)” or “Yes, with a template”
- Version control system: Git
Click the big blue button “Create repository.”
Copy the HTTPS or SSH clone URL that appears when you click on the blue “Clone” button.
Make sure you remove the git clone ...
that shows up at the beginning.
15.2 New RStudio Project via git clone
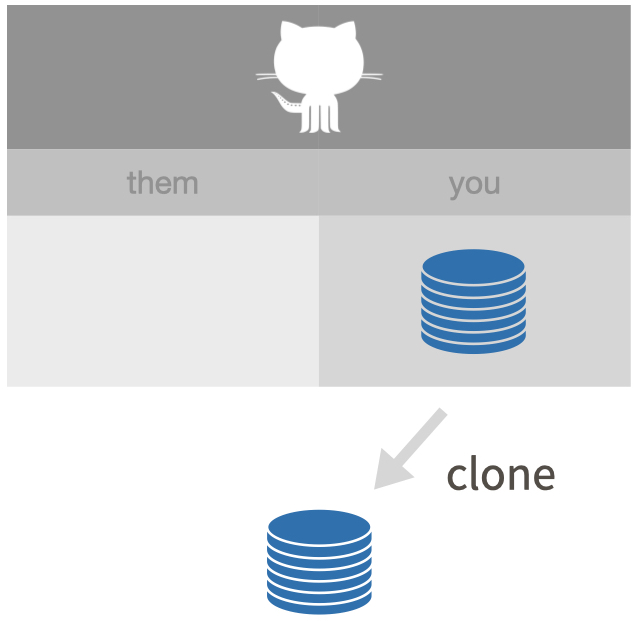
I present two ways to do this:
usethis::create_from_github()
- Via the RStudio IDE
(Recall that we showed how to do this with command line Git in chapter 11.)
When you are cloning your own GitHub repository, the two methods are equivalent.
In other scenarios, especially fork-and-clone (chapter 31), I think usethis::create_from_github()
is superior, because it does additional, recommended setup.
Pick one of these methods below.
15.2.1 usethis::create_from_github()
You can execute this command in any R session. If you use RStudio, then do this in the R console of any RStudio instance.
usethis::create_from_github(
"https://github.com/YOU/YOUR_REPO.git",
destdir = "~/path/to/where/you/want/the/local/repo/"
)
The first argument is repo_spec
and it accepts the GitHub repo specification in various forms.
In particular, you can use the URL we just copied from GitHub.
The destdir
argument specifies the parent directory where you want the new folder (and local Git repo) to live.
If you don’t specify destdir
, usethis defaults to some very conspicuous place, like your desktop.
If you like to keep Git repos in a certain folder on your computer, you can personalize this default by setting the usethis.destdir
option in your .Rprofile
.
We’re accepting the default behaviour of two other arguments, rstudio
and open
, because that’s what most people will want.
For example, for an RStudio user, create_from_github()
does this:
- Creates a new local directory in
destdir
, which is all of these things:- a directory or folder on your computer
- a Git repository, linked to a remote GitHub repository
- an RStudio Project
- Opens a new RStudio instance in the new Project
- In the absence of other constraints, I suggest that all of your R projects have exactly this set-up.
15.2.2 RStudio IDE
In RStudio, start a new Project:
-
File > New Project > Version Control > Git. In the “repository URL” paste
the URL of your new GitHub repository. It will be something like this
https://github.com/jennybc/myrepo.git
. - Be intentional about where you create this Project.
- I suggest you “Open in new session”.
- Click “Create Project” to create a new directory, which will be all of these things:
- a directory or “folder” on your computer
- a Git repository, linked to a remote GitHub repository
- an RStudio Project
- In the absence of other constraints, I suggest that all of your R projects have exactly this set-up.
This should download the README.md
file that we created on GitHub in the previous step.
Look in RStudio’s file browser pane for the README.md
file.
Behind the scenes, RStudio has done this for you:
git clone https://github.com/jennybc/myrepo.git
15.2.3 Have a look around
Regardless of whether you used usethis or RStudio, you should now be working in the new Git repo.
The implicit git clone
should download the README.md
file that we created on GitHub in the previous step.
Look in RStudio’s file browser pane for the README.md
file.
There’s a big advantage to the “GitHub first, then RStudio” workflow: the remote GitHub repo is configured as the origin
remote for your local repo and your local main
branch is now tracking the main
on GitHub.
This is a technical but important point about Git.
The practical implication is that you are now set up to push and pull.
No need to fanny around setting up Git remotes and tracking branches on the command line.
We’re about to confirm we are setup for pulling and pushing.
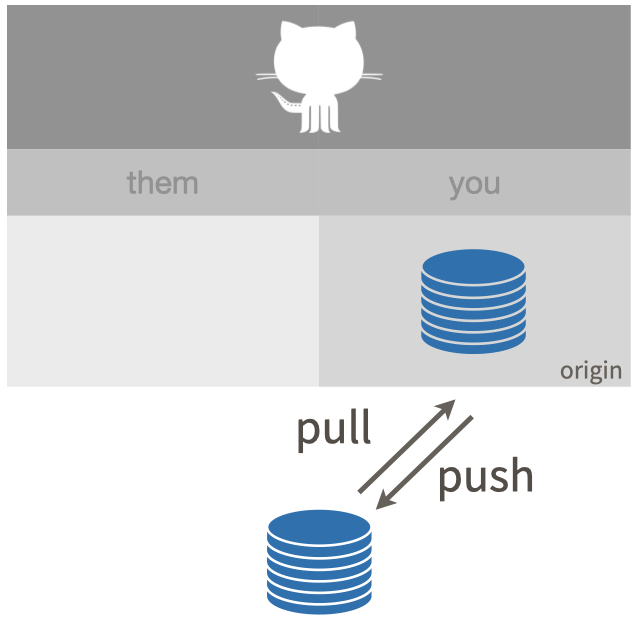
Figure 15.1: Pull and push.
15.2.4 Optional: peek under the hood
Completely optional activity: use command line Git to see what we’re talking about above, i.e. the remote and tracking branch setup.
git remote -v
or git remote --verbose
shows the remotes you have setup.
Here’s how that looks for someone using HTTPS with GitHub and calling it origin
:
~/tmp/myrepo % git remote -v
origin https://github.com/jennybc/myrepo.git (fetch)
origin https://github.com/jennybc/myrepo.git (push)
git branch -vv
prints info about the current branch (-vv
for “very verbose”, I guess).
In particular, we can see that local main
is tracking the main
branch on origin
, a.k.a. origin/main
.
~/tmp/myrepo % git branch -vv
* main 2899c91 [origin/main] A commit from my local computer
Finally, git remote show origin
gives yet another view on useful remote and branch information:
~/tmp/myrepo % git remote show origin
* remote origin
Fetch URL: https://github.com/jennybc/myrepo.git
Push URL: https://github.com/jennybc/myrepo.git
HEAD branch: main
Remote branch:
main tracked
Local branch configured for 'git pull':
main merges with remote main
Local ref configured for 'git push':
main pushes to main (up to date)
git clone
, which RStudio did for us, sets all of this up automatically.
This is why “GitHub first, then RStudio” is the preferred way to start projects early in your Git/GitHub life.
15.3 Make local changes, save, commit
Do this every time you finish a valuable chunk of work, probably many times a day.
From RStudio, modify the README.md
file, e.g., by adding the line “This is a line from RStudio”.
Save your changes.
Commit these changes to your local repo. How?
- Click the “Git” tab in upper right pane
- Check “Staged” box for any files whose existence or modifications you want to commit.
- To see more detail on what’s changed in file since the last commit, click on “Diff” for a Git pop-up
- If you’re not already in the Git pop-up, click “Commit”
- Type a message in “Commit message”, such as “Commit from RStudio”.
- Click “Commit”
15.4 Push your local changes to GitHub
Do this a few times a day, but possibly less often than you commit.
You have new work in your local Git repository, but the changes are not online yet.
This will seem counterintuitive, but first let’s stop and pull from GitHub.
Why? Establish this habit for the future! If you make changes to the repo in the browser or from another machine or (one day) a collaborator has pushed, you will be happier if you pull those changes in before you attempt to push.
Click the blue “Pull” button in the “Git” tab in RStudio. I doubt anything will happen, i.e. you’ll get the message “Already up-to-date.” This is just to establish a habit.
Click the green “Push” button to send your local changes to GitHub. RStudio will report something along these lines:
>>> /usr/bin/git push origin HEAD:refs/heads/main
To https://github.com/jennybc/myrepo.git
2899c91..b34cade HEAD -> main
15.5 Confirm the local change propagated to the GitHub remote
Go back to the browser. I assume we’re still viewing your new GitHub repo.
Refresh.
You should see the new “This is a line from RStudio” in the README.
If you click on “commits,” you should see one with the message “Commit from RStudio”.
15.6 Make a change on GitHub
Click on README.md in the file listing on GitHub.
In the upper right corner, click on the pencil for “Edit this file”.
Add a line to this file, such as “Line added from GitHub.”
Edit the commit message in “Commit changes” or accept the default.
Click the big green button “Commit changes.”
15.6.1 GitLab
Click on README.md in the file listing on GitLab.
In the upper right corner, click on “Edit”.
Add a line to this file, such as “Line added from GitLab.”
Edit the commit message in “Commit changes” or accept the default.
Click the big green button “Commit changes.”
15.6.2 Bitbucket
Click on README.md in the file listing on Bitbucket.
In the upper right corner, click on “Edit”.
Add a line to this file, such as “Line added from Bitbucket.”
Click on the blue “Commit” button. A pop-up will show up. Edit the commit message or accept the default.
Click the blue “Commit” button.
15.7 Pull from GitHub
Back in RStudio locally …
Inspect your README.md. It should NOT have the line “Line added from GitHub”. It should be as you left it. Verify that.
Click the blue Pull button.
Look at README.md again. You should now see the new line there.
15.8 The end
Now just “lather, rinse, repeat”. Do work somewhere: locally or on GitHub. Commit it. Push it or pull it, depending on where you did the work, but get local and remote “synced up”. Repeat.
Note that in general (and especially in future when collaborating with other developers) you will usually need to pull changes from the remote (GitHub) before pushing the local changes you have made. For this reason, it’s a good idea to try and get into the habit of pulling before you attempt to push.